Halliday Payments vs Reactive
This article compares Halliday’s off-chain, SDK-based approach to payments and onboarding with Reactive Network’s fully on-chain, event-driven architecture—highlighting how each serves different ends of the Web3 spectrum: user-friendly commerce vs. protocol-level automation.
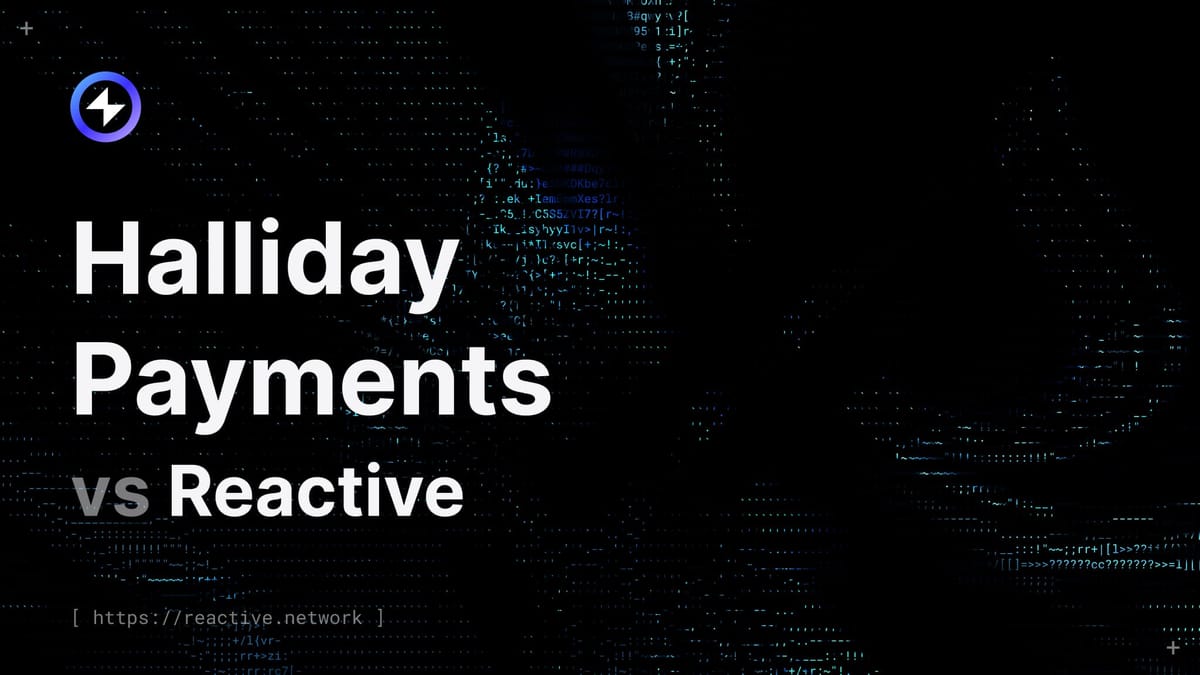
Halliday is a commerce infrastructure platform for Web3. It allows users to acquire tokens on any supported blockchain with minimal setup. Built with a self-custodial architecture, Halliday ensures that users retain full control over their assets throughout all transactions.
The platform provides a suite of services, including fiat on-ramps and off-ramps, cross-chain swaps, and integrations with major exchanges — enabling direct payments across networks without the need for intermediaries.
Solution
Developers building in Web3 often face challenges related to fragmented infrastructure and complex user flows. One common issue is the fragmented user experience — users typically rely on several tools to move assets across different blockchains. Halliday addresses this with a unified interface that handles operations across chains in a consistent way.
Another challenge is the high development overhead. Without Halliday, developers must integrate a range of services, including bridges, decentralized exchanges, and payment providers, each with its own API and maintenance requirements. Halliday simplifies this process by offering a single API that consolidates this functionality.
Finally, user onboarding can be unnecessarily complicated. Acquiring tokens on specific chains often involves multiple steps, which increases drop-off and support load. Halliday reduces this complexity by supporting direct fiat-to-token purchases on any supported network.
Integration
To integrate Halliday into your application, follow these steps:
Step 1 – Obtain API Keys
Begin by creating an account on the Halliday Dashboard. From there, generate API keys for both sandbox (testing) and production environments. These keys will be required to authenticate calls to the SDK.
Step 2 – Install the SDK
Install the commerce SDK via npm: npm install @halliday-sdk/commerce
Step 3 – Select an Integration Method
Depending on your use case, you can choose from several integration patterns:
Basic Popup with Onramp ServiceA simple button that opens the Halliday interface to purchase tokens using fiat.
Embedded Widget with Onramp Service
Renders the onramp flow inside a specified DOM container. Useful for maintaining layout consistency.
Popup Widget with Swap Service
Opens a modal that enables users to swap tokens. Requires wallet connection.
Combined Onramp & Swap Services
Enables both fiat purchases and token swaps from a single entry point.
Additional options include:
Custom Styling
You can apply custom styles to match your application’s design language when embedding widgets.
Headless Integration for Custom UI
Developers looking to build fully customized flows can use Halliday’s headless client. This gives complete control over UI while relying on Halliday for core functionality like routing, quoting, and settlement.
Key Benefits
Reduced Development Time
Integrating Halliday takes hours instead of weeks. Developers no longer need to build and maintain separate connections to bridges, DEXs, fiat providers, and wallets. A single integration replaces multiple service-specific implementations.
Simplified Maintenance
Halliday consolidates various services behind a unified API. This reduces long-term maintenance costs, as updates to individual providers are managed internally by Halliday — removing the burden of keeping up with breaking changes or service migrations.
Improved User Experience
Halliday enables direct token acquisition and cross-chain transactions. Fewer steps mean fewer points of failure, reducing user drop-off and increasing conversion rates across onboarding and transaction flows.
Understanding Reactive Contracts
Reactive contracts are engineered for real-time, event-based automation entirely on-chain, with a particular strength in cross-chain scenarios. Instead of relying on off-chain schedulers or interval-based checks, Reactive contracts directly monitor blockchain events and respond immediately — enabling fast, predictable execution across networks.
They’re especially well-suited for developers who require complete on-chain control, cross-chain orchestration, and the ability to compose logic across contracts — all without external dependencies.
The core principles are:
- Reactive Triggers: Native on-chain listeners that activate contract logic in response to live events
- Cross-Chain Execution: Built-in support for routing actions across origin and destination chains
- Composable Architecture: Connect modular pieces of logic without altering base contracts
- Deterministic Automation: Every action is verifiable and reproducible directly on-chain
Feature Comparison
Feature | Reactive Contracts | Halliday Payments |
---|---|---|
Automation Type | On-chain, event-triggered | Off-chain SDK/API automation |
Cross-Chain Support | ✅ Native event-based routing | ✅ Unified flow across chains |
UI & Dev Experience | ⚠️ Requires contract deployment | ✅ SDK, popup & widget UIs |
Execution Environment | Solidity-native | JS/TS SDK + smart contracts |
Determinism & Traceability | ✅ Fully on-chain & traceable | ⚠️ Off-chain flow, contract-visible |
Custody Model | On-chain, contract-based | Self-custodial (user holds keys) |
Ideal For | Protocol automation, governance | Onboarding, commerce, fiat onramp |
Recap
Halliday is a commerce-focused SDK platform enabling dApps to onboard users easily, offer fiat-to-token payments, and execute cross-chain token flows through an API and customizable UI components. It excels in improving UX and reducing integration overhead — perfect for teams prioritizing onboarding, payments, or multi-chain asset access.
Reactive, on the other hand, is optimized for protocol-native automation — where deterministic, event-driven logic must run entirely on-chain. It shines in use cases where security, transparency, and composability are non-negotiable — such as DAO governance, cross-chain DeFi flows, or decentralized interchain coordination.
If your goal is to build trustless systems that automate logic at the protocol layer, choose Reactive. If you’re building a user-friendly, payment-enabled dApp, Halliday provides the fastest and most developer-friendly path to production.
About Reactive Network
The Reactive Network, pioneered by PARSIQ, ushers in a new wave of blockchain innovation through its Reactive Smart Contracts (RSCs). These advanced contracts can autonomously execute based on specific on-chain events, eliminating the need for off-chain computation and heralding a seamless cross-chain ecosystem vital for Web3’s growth.
Central to this breakthrough is the Inversion of Control (IoC) framework, which redefines smart contracts and decentralized applications (DApps) by imbuing them with unparalleled autonomy, efficiency, and interactivity. By marrying RSCs with IoC, Reactive Network is setting the stage for a transformative blockchain era, characterized by enhanced interoperability and the robust, user-friendly foundation Web3 demands.
Website | Blog | Twitter | Telegram | Discord | Docs