Reactive Bridge: Decentralizing Cross-Chain Token Transfers
Reactive Bridge enables secure, decentralized token transfers across blockchains by automating cross-chain transactions through event-driven smart contracts, removing reliance on intermediaries. This scalable solution enhances cross-chain interoperability for DeFi and Web3 ecosystems.
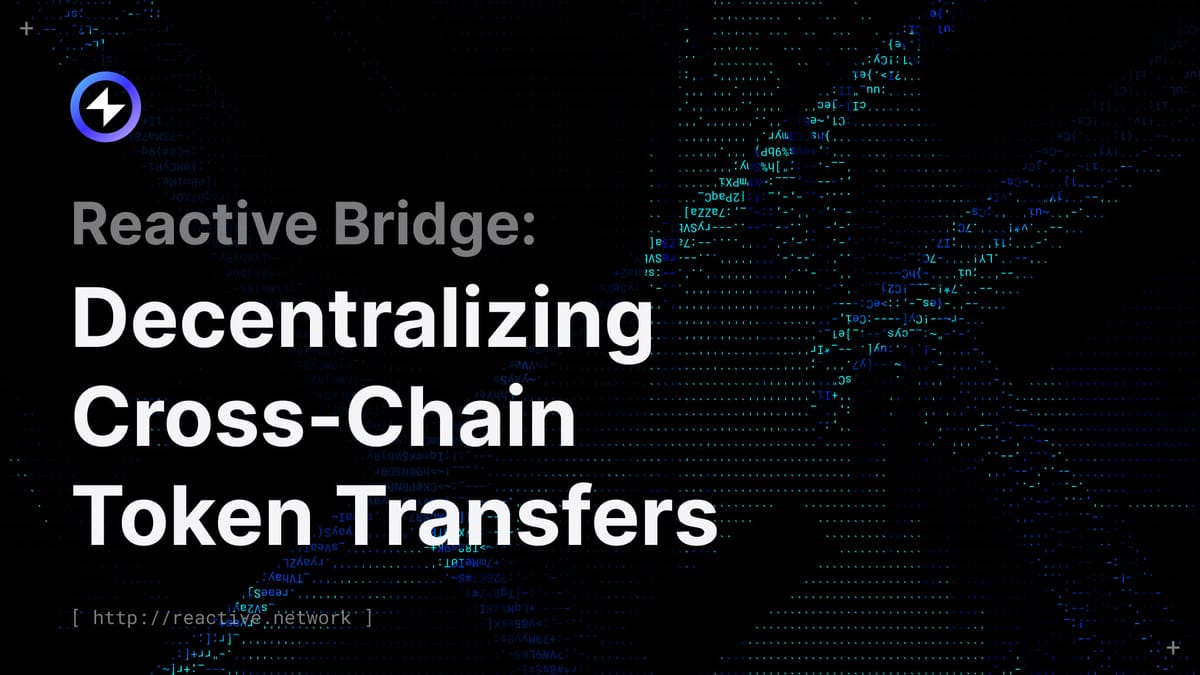
Introduction
In a multi-chain ecosystem, interoperability is key for asset transfers. Reactive Bridge leverages smart contracts and event-driven architecture to securely transfer tokens across different blockchains. This article explores its implementation, drawing from the code of Salman Dabbakuti, the Reactive Hackathon Bi-Weekly-Challenge winner on DoraHacks. It demonstrates how the system reacts to events on the origin chain and triggers minting on the destination chain.
We extend our gratitude to Salman for his contribution to advancing cross-chain token bridging technology. You can also check out the Reactive Bridge Demo or test Reactive Bridge yourself.
Why Reactive Bridge Matters
Traditional token bridges often rely on intermediaries or validators, introducing trust and potential centralization risks. Reactive Bridge mitigates these concerns by using reactive smart contracts, reducing reliance on centralized validators. This trust-minimized, decentralized approach enhances security, scalability, and efficiency in dApps, DeFi, and cross-chain protocols.
Key Features
Two-way Token Bridge: Reactive Bridge supports bidirectional token transfers between two chains (e.g., A↔B). The reactive contract listens for events on both chains and triggers token minting on the destination chain. The current demo focuses specifically on transfers from Polygon (A) to Ethereum Sepolia (B).
Intuitive UI: The demo features a user-friendly interface (similar to Uniswap's Swap UI) for initiating bridge transfers. Users can enter the token amount to request a transfer between chains.
Real-time Updates: The UI updates token balances in real time, providing a clear view of the entire bridge transfer process, from the request to the minting of tokens on the destination chain.
Implementation
Reactive Bridge is powered by two smart contracts that coordinate cross-chain transfers:
CrossToken (Origin/Destination Chain): This ERC-20 token contract, deployed on both the origin and destination chains (`origin1`, `origin2`), emits a `BridgeRequest` event whenever a user requests a token transfer. This event contains the user’s address and the token amount.
ReactiveBridge (Reactive Network): Monitoring the origin chain for `BridgeRequest` events, this contract reacts by minting tokens on the destination chain. It follows an event-driven, reactive architecture, ensuring cross-chain actions are automatically synchronized.
Token Bridging
The `CrossToken` contract facilitates the creation and bridging of tokens. When a user wants to send tokens to another chain, they initiate a `bridgeRequest`. This request burns the tokens on the origin chain, and the corresponding amount is minted on the destination chain by the `ReactiveBridge` contract.
function bridgeRequest(uint256 _amount) external {
_burn(msg.sender, _amount);
emit BridgeRequest(address(this), block.chainid, msg.sender, _amount);
}
Reactive Event Handling
The `ReactiveBridge` contract subscribes to events from the `CrossToken` contract on the origin chain. When a `BridgeRequest` event is detected, the `react()` function is triggered to handle the bridging process. It decodes the receiver and amount from the event data and emits a `Callback` to the destination chain to mint the equivalent tokens.
function react(
uint256 chain_id,
address _contract,
uint256 topic_0,
uint256 topic_1,
uint256 topic_2,
uint256 topic_3,
bytes calldata data
) external vmOnly {
(, , address receiver, uint256 amount) = abi.decode(
data,
(address, uint256, address, uint256)
);
bytes memory payload = abi.encodeWithSignature(
"bridgeMint(address,address,uint256)",
address(0),
receiver,
amount
);
uint256 destinationChainId = chain_id == origin1ChainId ? origin2ChainId : origin1ChainId;
address destination = _contract == origin1 ? origin2 : origin1;
emit Callback(destinationChainId, destination, GAS_LIMIT, payload);
}
Multi-Chain Support
Reactive Bridge supports bridging between multiple chains by maintaining reverse mappings between the origin and destination chains. This allows the contract to react to events on one chain and propagate actions to another.
uint256 destinationChainId = chain_id == origin1ChainId ? origin2ChainId : origin1ChainId;
address destination = _contract == origin1 ? origin2 : origin1;
emit Callback(destinationChainId, destination, GAS_LIMIT, payload);
Callbacks
Callbacks are essential for bridging operations. They ensure that the correct function calls are executed on the destination chain based on events triggered on the origin chain, decoupling the bridging logic from specific chains and improving flexibility and scalability.
For example, when a `BridgeRequest` event is triggered on the origin chain, the following callback is emitted to the destination chain:
bytes memory payload = abi.encodeWithSignature(
"bridgeMint(address,address,uint256)", // function to mint tokens
address(0), // ReactVM address
receiver, // recipient of the minted tokens
amount // amount to mint
);
emit Callback(destinationChainId, destination, GAS_LIMIT, payload);
In this case, the `Callback` event contains the function signature, recipient address, and amount to mint on the destination chain. This mechanism allows the bridge to function independently of the chain specifics.
Conclusion
Reactive Bridge presents a decentralized solution for cross-chain token transfers, removing the need for centralized intermediaries. By utilizing reactive smart contracts and an event-driven architecture, it provides a foundation for cross-chain DeFi, dApp ecosystems, and broader blockchain interoperability.
About Reactive Network
The Reactive Network, pioneered by PARSIQ, ushers in a new wave of blockchain innovation through its Reactive Smart Contracts (RSCs). These advanced contracts can autonomously execute based on specific on-chain events, eliminating the need for off-chain computation and heralding a seamless cross-chain ecosystem vital for Web3’s growth.
Central to this breakthrough is the Inversion of Control (IoC) framework, which redefines smart contracts and decentralized applications (DApps) by imbuing them with unparalleled autonomy, efficiency, and interactivity. By marrying RSCs with IoC, Reactive Network is setting the stage for a transformative blockchain era, characterized by enhanced interoperability and the robust, user-friendly foundation Web3 demands.